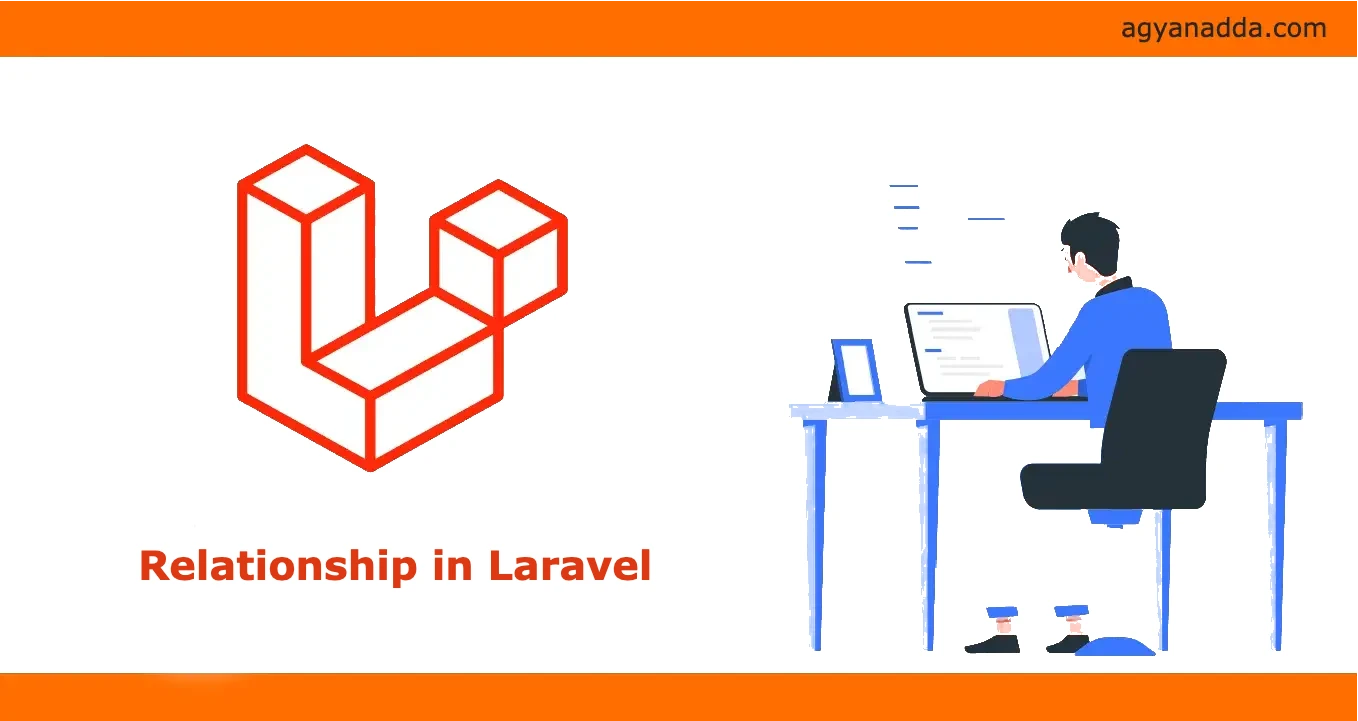
What is Query Builder and Eloquent in Laravel
Query Builder and Eloquent in Laravel
The Query Builder and Eloquent is most important part of...
Query Builder and Eloquent in Laravel
The Query Builder and Eloquent is most important part of...
In Laravel, service providers are the central place to configure and bootstrap application services. It...
In Laravel, we can optimize the speed and performance by using some techniques. There are...
Oops stands for object-oriented programming language. It is also known as a procedural programming language....
In bootstrap when you want to use Modal to show any message then it is...
In Laravel we will learn about Traits and how to upload image in folder using...
In this article we will learn about log query in Laravel. It helps us to...
In Laravel we will learn how to add new column to existing table without...
In this article, we will learn how to upload an image in React and Node...
What is Query Builder and Eloquent in Laravel
What is service provider in laravel
How to Optimize the Speed & Performance of a Laravel Website
Auto refresh code in HTML using meta tags
How to disable click outside modal to close modal in bootstrap
What is traits in PHP with example
Laravel Eloquent display query log in php
How to add new column to existing table without losing data in Laravel